1. Installation
Before starting, make sure to install tkinter in your system
sudo apt update
sudo apt install python3-tk
2. Create Window
import tkinter as tk
# Create the main window
root = tk.Tk()
# Window title
root.title("Simple GUI")
# Window Size (width x height)
root.geometry("400x300")
3. Create Label
# Create a label
label = tk.Label(root, text="Hello, World!")
# Add the label to the window with vertical padding
label.pack(pady=20)
4. Create a Button
# Create a close button
close_button = tk.Button(root, text="Close", command=root.quit)
# Add vertical padding
close_button.pack(pady=20)
5. Full Code
import tkinter as tk
# Create the main window
root = tk.Tk()
# Window title
root.title("Simple GUI")
# Window Size (width x height)
root.geometry("400x300")
# Create a label
label = tk.Label(root, text="Hello, World!")
# Add the label to the window with vertical padding
label.pack(pady=20)
# Create a close button
close_button = tk.Button(root, text="Close", command=root.quit)
# Add vertical padding
close_button.pack(pady=10)
# Run the main loop
root.mainloop()
6. Output
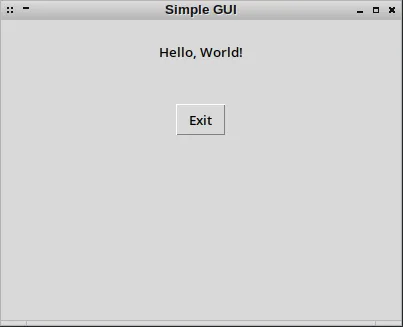